Learn how poorly written SQL queries can severely degrade database performance, leading to slow response times and inefficient resource utilization.
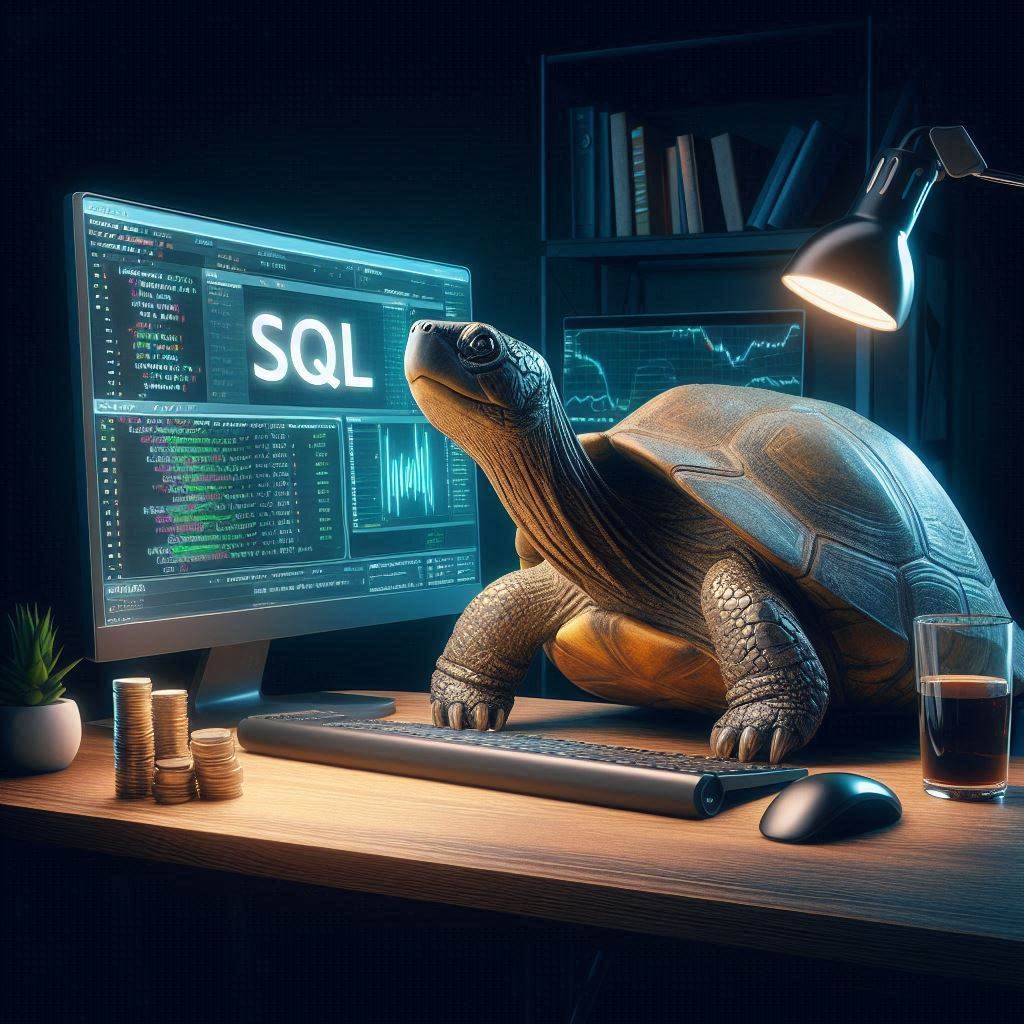
Table of Contents
Introduction
Imagine you’re working on a project with a heavy dataset. This project’s primary task is to fetch data and compare results. In another service, It involves complex calculations on large datasets. In both cases, to achieve optimal performance, you rely heavily on native SQL queries. Initially, these queries might seem manageable and straightforward. However, maintaining these SQL queries becomes increasingly challenging as the project evolves and the data grows.
Over time, what once were clear and efficient queries can become cumbersome and difficult to understand. The complexity of the queries increases as new requirements are added, and the lack of documentation or comments escalates the problem. Furthermore, different developers might contribute to the SQL codebase, each with their own style and level of expertise, leading to inconsistencies and potential inefficiencies.
As a result, these SQL queries can become hard to read, maintain, and optimize. This complexity not only impacts the development team’s productivity but also the performance and scalability of the system. Without regular review and optimization, bad SQL queries can lead to significant performance degradation, making the application slower and more resource-intensive over time.
What is a Bad SQL Query
A bad SQL query is inefficient, poorly optimized, or incorrectly written, leading to performance issues, increased resource consumption, and potential scalability problems. These queries may fetch more data than necessary, use inefficient joins, lack proper indexing, or be written in a way that causes unnecessary complexity.
Common Mistakes to Avoid When Writing SQL Queries:
Using
SELECT *
:Issue: Fetches all columns, leading to unnecessary data transfer and slower performance.
Solution: Specify only the required columns.
SELECT column1, column2 FROM table;
Full Table Scans:
Issue: Occurs when queries lack appropriate indexes, leading to slow performance.
Solution: Ensure columns in WHERE clauses are indexed.
CREATE INDEX idx_column ON table(column);
Leading Wildcards in LIKE Clauses:
Issue: Prevents index usage, resulting in full table scans.
Solution: Avoid leading wildcards in LIKE conditions.
WHERE column LIKE 'value%'
Ignoring Execution Plans:
Issue: Not analyzing execution plans can lead to undetected performance issues.
Solution: Regularly use EXPLAIN
or EXPLAIN ANALYZE
to understand query performance.
EXPLAIN ANALYZE SELECT * FROM table WHERE condition;
Inefficient Joins:
Issue: Using joins without indexes or poorly designed join conditions can degrade performance.
Solution: Index join columns and use appropriate join types. By focusing on the primary join condition and ensuring the relevant columns are indexed, you can achieve better query performance.
SELECT * FROM Orders o JOIN Customers c ON o.CustomerID = c.CustomerID;
Lack of Pagination:
Issue: Fetching large result sets without limits can overwhelm the database and application.
Solution: Implement pagination using LIMIT and OFFSET.
SELECT * FROM Products ORDER BY Price LIMIT 20 OFFSET 0;
Ease of Writing Bad Queries
SQL’s easy-to-use language and simple structure make it accessible but also lead to the creation of slow and inefficient queries. Many users, especially those without a deep understanding of database internals, can write queries that look correct but perform poorly. This is because SQL allows different ways to get the same result, and some methods are much less efficient. Common problems like poor indexing, selecting too much data, and using inefficient joins often arise, causing the database to run much slower.
Common Reasons for Writing Inefficient SQL Queries:
Lack of Knowledge:
Developers may need to be fully aware of best practices for writing efficient SQL queries, such as the importance of indexing, avoiding SELECT *, and proper join strategies.
Complex Requirements:
When requirements are complex, it can be easy to write convoluted or overly complex queries that perform poorly.
Tight Deadlines:
Under tight deadlines, developers might prioritize functionality over performance, leading to suboptimal queries.
Insufficient Tools:
Without proper tools for query analysis and optimization, developers might not recognize performance issues in their queries.
Overreliance on ORMs:
While Object-Relational Mappers (ORMs) simplify database interactions, they can sometimes generate inefficient queries, and developers may not realize it.
Changing Data Patterns:
Queries written based on initial data patterns might become inefficient as the dataset grows or changes, but these queries may not be revisited or optimized accordingly.
The ease of writing bad queries highlights the need for continuous learning, code reviews, and performance analysis tools to ensure that SQL queries remain efficient and maintainable.
Situations Where the System Can Live with Bad Queries
While optimizing SQL queries is generally best practice, certain scenarios allow a system to tolerate inefficient queries without significant negative impact.
Here are some situations where this is feasible:
Small Dataset:
The application deals with a small volume of data. Even inefficient queries execute quickly because the dataset is small, minimizing the performance hit.
Low Query Frequency
The problematic queries are run infrequently. Since these queries don’t run often, the system isn’t slowed down by them, allowing it to maintain overall performance.
Non-Critical Applications: The application is not mission-critical (e.g., internal tools, low-priority projects).
Performance issues are more tolerable as they don’t impact key business operations or user satisfaction significantly.
Plenty of System Resources: The system has more than enough computational power (CPU, memory, I/O capacity).
Extra resources can handle inefficiencies, avoiding noticeable slowdowns.
Effective Caching: The application employs robust caching strategies.
Caching reduces the load on the database by serving repeated queries from the cache, mitigating the impact of inefficient queries.
Background Processing: Inefficient queries run as part of batch jobs or background tasks.
Since these queries do not affect real-time user interactions, their inefficiency is less critical.
Development and Testing Phases: The application is in the development or testing phase.
Performance optimization can be deferred until the application is closer to production, allowing developers to focus on functionality first.
Redundant Architecture: The system architecture includes redundancy, such as load balancing or failover mechanisms.
Redundancy ensures system availability and mitigates the impact of inefficient queries by distributing the load.
Low User Concurrency: The application has a low number of concurrent users or queries.
Low concurrency reduces the likelihood of performance bottlenecks, as the system can handle inefficient queries more easily.
Strategic Considerations
While these scenarios allow for some tolerance of inefficient queries, it’s still important to:
- Monitor System Performance: Keep an eye on performance metrics to catch any emerging issues early.
- Plan for Optimization: Gradually optimize inefficient queries, especially as the dataset grows or usage patterns change.
- Ensure Scalability: Regularly review and refactor queries to prepare for future scalability and increased load.
While these scenarios allow a system to function effectively despite inefficient queries, it remains beneficial to address and optimize these queries over time to ensure future scalability and performance.
Unnoticed Issues from Bad Queries
While inefficient SQL queries might not immediately appear problematic, they can lead to a range of issues that may go unnoticed until they become significant. Here are some potential problems caused by bad queries:
Gradual Performance Degradation: Over time, inefficient queries can cause the overall performance of the application to degrade and users may experience increasingly slower response times, which can affect user satisfaction and productivity.
Increased Resource Consumption: Bad queries often consume more CPU, memory, and I/O resources than necessary. This can lead to higher operational costs and strain on system resources, potentially affecting other applications running on the same infrastructure.
Hidden Bottlenecks: Inefficient queries can create hidden performance bottlenecks that are not immediately apparent. These bottlenecks can affect scalability and become critical issues as the application grows or user load increases.
Hidden Scalability Issues: Bad queries can make it hard for the application to grow. When more data or users are added, the application might have trouble working well.
Reduced Throughput: Bad queries can cause resource conflict, where multiple processes compete for the same resources. This can lead to increased latency and reduced performance for all database operations.
Delayed Maintenance Windows: Inefficient queries can extend the time required for routine maintenance tasks like backups, indexing, or data migrations. Prolonged maintenance windows can lead to increased downtime and impact service availability.
Data Inconsistency: Complex or poorly written queries may result in data inconsistency or inaccuracies if not carefully managed. This can compromise data integrity, leading to incorrect reporting and decision-making.
Increased Debugging and Troubleshooting Time
Identifying and fixing issues caused by bad queries can be time-consuming and complex.
This increases the maintenance overhead and can delay the resolution of other critical issues.
Suboptimal User Experience
Users may experience occasional slowdowns or unpredictable performance. Poor user experience can lead to decreased user satisfaction and potential loss of customers or users.
By proactively addressing these unnoticed issues, you can maintain optimal system performance, improve user satisfaction, and avoid costly fixes in the future.
Importance of Writing Efficient SQL Queries from the Start
Starting with efficient SQL queries is crucial for maintaining high performance in your database systems. Early optimization ensures that your queries run quickly and efficiently, reducing the load on your system and improving the overall user experience.
Once inefficient queries are in place and your application grows, it becomes increasingly difficult and costly to refactor and optimize them. Implementing best practices from the beginning, such as proper indexing, selective data retrieval, and efficient joins, helps avoid performance bottlenecks and scalability issues down the line.
By focusing on writing efficient queries initially, you can ensure your system remains responsive and robust, supporting smooth growth and adaptation to increasing data volumes and user demands.
Optimizing SQL Queries for Performance Efficiency and Cost Management
When writing SQL queries, it’s crucial to understand the factors that impact performance efficiency and the associated costs.
Here’s a detailed description to guide you through these concepts:
Query Performance Efficiency:
refers to how well a SQL query utilizes database resources (CPU, memory, disk I/O) to retrieve the desired results. Efficient queries execute quickly and use minimal resources, which is essential for maintaining a responsive and scalable database system.
Cost of SQL Queries:
The cost of SQL queries encompasses the computational and resource expenses incurred when executing a query. This includes CPU time, memory usage, and disk I/O operations. High-cost queries can lead to slower performance, increased operational costs, and can affect the performance of other queries running on the same system.
Key components influencing the cost of SQL queries include:
- CPU Time: Complex calculations and functions increase the CPU time required to process a query.
- Memory Usage: Queries that process large datasets or require significant sorting and joining operations consume more memory.
- Disk I/O: Queries that need to read or write large amounts of data from disk can be costly in terms of I/O operations.
- Network Latency: In distributed database systems, data retrieval across networked nodes can add to the query cost.
Tools to check SQL query performance
Explain Plans
Provides insights into how the database executes a query.
Supported by MySQL, PostgreSQL, Oracle, and SQL Server.
Helps identify query execution paths and potential bottlenecks.
Database-Specific Tools
- MySQL Workbench: Graphical tool for query performance visualization.
- MySQL Performance Schema: Monitors and analyzes query performance metrics.
- pgAdmin: Offers query planning and execution insights.
- pgBadger: Analyzes PostgreSQL logs for performance reports.
- SQL Server Management Studio (SSMS): Provides graphical query plans.
- SQL Profiler: Tool for tracing and analyzing SQL queries.
- SQL Developer: Detailed execution plans and tuning advisors.
- AWR (Automatic Workload Repository): Collects performance statistics.
Third-Party Tools
New Relic: Application performance monitoring with SQL query analysis. Identifies and optimizes slow queries.
Dynatrace: Real-time monitoring and analytics for SQL queries. Tracks query performance across the application stack.
IDE Plugins
JetBrains DataGrip: Database IDE with query execution plans and performance analysis.
SQL Server Data Tools (SSDT): Integrated with Visual Studio, provides SQL Server query tuning tools.
Digma: An observability tool created to offer comprehensive insights into different aspects of your codebase.
Cloud Provider Tools
AWS RDS Performance Insights: Performance monitoring and tuning for Amazon RDS databases.
Google Cloud SQL Insights: Query performance analyzer for Google Cloud SQL databases.
Azure SQL Database Advisor: Recommends performance improvements for Azure SQL databases.
Using these tools, you can effectively monitor, analyze, and optimize the performance of your SQL queries across various database platforms, ensuring efficient operation and scalability of your applications.
How Digma can provide insights into query optimization
Digma is a platform for Continuous Feedback that seamlessly integrates observability into the development workflow. It aims to offer developers immediate insights into their code’s performance, scalability, and potential issues directly within their IDE. This approach enables developers to detect and resolve issues early in the development process, improving code quality and reducing challenges during production.
Requirements
- Set up your spring boot application
- Add the Digma plugin to your development environment.
- Add observability to your code
In this article, we will use the petclinic sample code.
Let’s look at Digma’s insights to get feedback on implemented SQL queries.
Query Optimization
This feature identifies queries with low performance.
Digma analyzes database query traces to find common issues such as N+1 Selects queries (queries that have repeated select statements due to inefficient ORM modeling), bulk inserts optimizations, Open Session in view, and other issues and anti-patterns.
We call API :PetClinic:HTTP GET /owners
In the “Insight” tab, you can find all calls and traces. Icons appear for different insights.
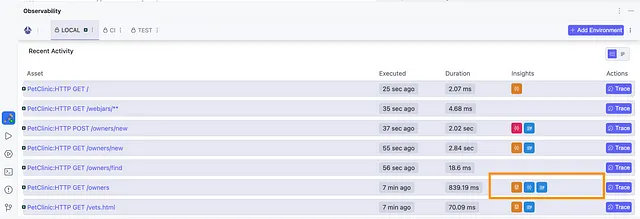
Provided insight highlights an inefficient SQL query detected by the continuous feedback system.

The query’s duration is significantly longer (7 milliseconds) compared to the typical duration of similar SELECT requests (434.61 microseconds).
This inconsistency indicates potential performance issues. The query in question retrieves distinct columns from the ‘owners’ table, impacting the HTTP GET /owners endpoint.
The feedback suggests that this query is an outlier in terms of performance, prompting developers to investigate and optimize it to align with expected performance standards, thereby improving overall system efficiency.
Integrating an IDE plugin that provides insights into low-performance SQL queries offers several advantages. It gives immediate feedback, allowing developers to correct inefficient queries in real-time, improving overall query performance. The plugin enhances developer productivity by automating query optimization and ensuring consistent code quality across the team.
Proactively identifying performance issues helps in maintaining system reliability and scalability, while also reducing infrastructure costs. Additionally, it serves as an educational tool, assisting developers to learn and adopt best practices for writing efficient SQL queries.
Conclusion
Writing efficient SQL queries is crucial for maintaining optimal performance, efficiency, and cost-effectiveness. While it’s easy to fall into the trap of writing bad queries, being aware of the common traps and actively working to understand and optimize SQL can mitigate these issues. Regularly analyzing query performance, understanding the database schema, and utilizing tools like execution plans can significantly improve the quality of your SQL queries, leading to a more robust and cost-effective system.
Common questions
- How can indexing improve the performance of SQL queries, and what are the best practices for using indexes?
Indexing improves SQL query performance by reducing search space, enabling faster data retrieval, optimizing join operations, and efficiently sorting/filtering data. Best practices include indexing columns used in WHERE, JOIN, ORDER BY, and GROUP BY clauses, using composite indexes, avoiding over-indexing, and regularly monitoring index usage. Proper indexing significantly enhances query efficiency and overall database performance.
2. How are SQL query speed and scalability connected?
SQL query speed and scalability go hand in hand in databases. When you run SQL queries efficiently, it helps your system handle more data or users without slowing down. If queries are slow or inefficient, they can make it harder for your system to grow smoothly as more people use it or more data is added. By making sure queries are optimized — like making them run faster and use fewer resources — you can make your system faster and ready for more users or data.
3. How can the readability and maintainability of SQL queries be improved while ensuring they remain efficient?
To improve the readability and maintainability of SQL queries while ensuring efficiency, use clear and descriptive aliases for tables and columns, and format queries with proper indentation. Break complex queries into manageable subqueries or use Common Table Expressions (CTEs). Add comments to explain non-obvious logic, and consistently follow naming conventions and best practices. Regularly review and refactor queries for simplicity and clarity without sacrificing performance.