The importance of indexes in optimizing database performance
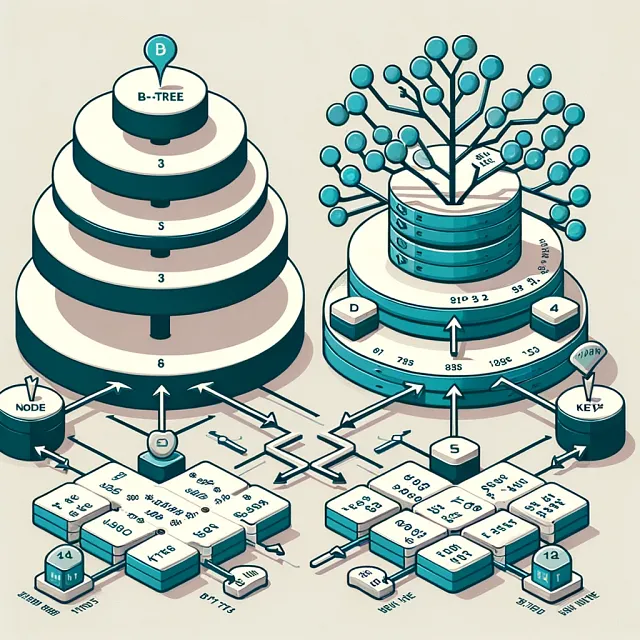
When managing a database, speed and efficiency are crucial. As applications handle more data and become more complex, the performance of database queries plays a big role in keeping everything running smoothly. One of the best ways to make queries faster is by using indexes. Similar to a book’s index that helps you quickly find a topic, database indexes allow you to find specific data without searching through the entire database. This article explains the basics of indexing, how it improves query performance, and some simple tips for using indexes effectively. Whether your database is small or large, understanding how to use indexes can help keep your application fast and responsive.
Table of Contents
What Are Indexes?
Indexing is a technique used in database management systems to improve the speed and efficiency of data retrieval operations. An index is a data structure that provides a quick way to look up rows in a table based on the values in one or more columns. Technically, an index is a data structure (usually a B-tree or a hash table) that stores the values of one or more columns in a way that allows for quick searches, sorting, and filtering.
The index provides pointers to the actual rows in the database table where the data resides. For example, if many queries filter or sort by a specific column, indexing that column can improve performance.
Types of Indexes
1. Primary Index: Automatically created when a primary key is defined. It uniquely identifies each row in the table.
To define a primary index in a Spring Boot application, you need to annotate the primary key field in your entity class with the @Id
annotation. This indicates that the field is the primary key, and most relational databases will automatically create an index on this column to optimize searches based on the primary key.
2. Secondary Index: Created on columns that are not unique or are frequently used in queries for filtering or sorting.
@Table(indexes = @Index(...))
: This creates a secondary index on the email
column with the name idx_user_email
. This index will speed up queries that filter or sort by the email
field.
@Entity @Table( name = "users", indexes = @Index(name = "idx_user_email", columnList = "email") ) public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private String email; // Getters and Setters }
3. Clustered Index: Sorts and stores the data rows of the table based on the index key. A table can have only one clustered index because the data rows can be sorted in only one order.
In many databases, the primary key is clustered by default, so if you want to cluster on a different column, you would need to use a specific SQL statement during database setup or use a custom annotation if your database supports it.
Custom SQL to Create a Clustered Index
For a clustered index on a non-primary key column, you’d usually execute a SQL script. Here’s how you might do it for a MySQL database:
CREATE TABLE users ( id BIGINT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), email VARCHAR(255) ); CREATE INDEX idx_users_name ON users(name); ALTER TABLE users DROP PRIMARY KEY, ADD PRIMARY KEY(name);
You can run this SQL script during your application startup using a schema.sql
file in your resources
directory, or manually in your database.
4. Non-clustered Index: Contains a sorted list of values along with pointers to the data rows where the values are found. A table can have multiple non-clustered indexes. A table can have multiple non-clustered indexes.
Create a Non-Clustered Index on the name
column in a User
entity.@Table(indexes = @Index(...))
: This annotation creates a non-clustered index on the name
column, which will improve the performance of queries that filter or sort by the name
field.
@Entity @Table( name = "users", indexes = @Index(name = "idx_user_name", columnList = "name") ) public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private String email; // Getters and Setters }
These examples show how to create and use different types of indexes within a Spring Boot application using JPA, but the exact implementation can vary depending on the database system you’re using.
Indexing Benefits
How Indexing Improves Query Performance
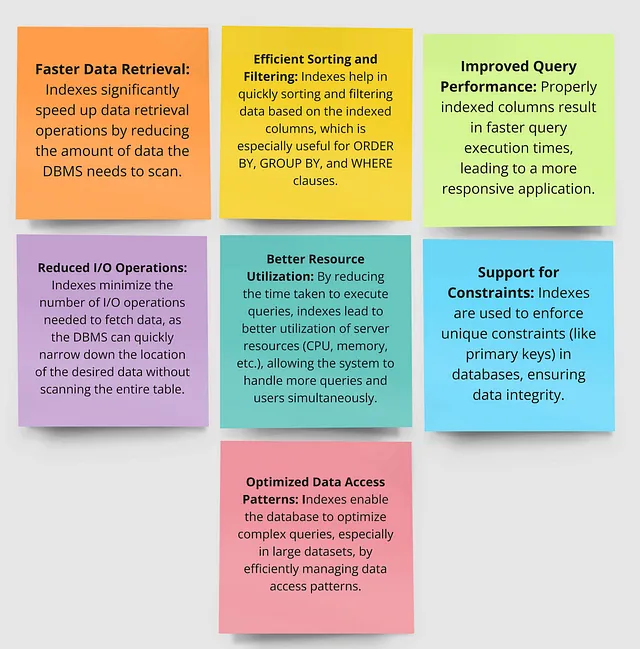
How Indexing Improves Query Performance
Implementation Considerations
Understanding When and Why to Implement Indexes for Maximum Efficiency
Using indexes in a database can significantly improve query performance, but it’s essential to understand when and why you should create them.
Here are the key conditions under which you should consider using indexes:
1. High-Volume Read Operations
Tables that are frequently queried for data retrieval, especially in read-heavy applications. Indexes speed up data retrieval by allowing the database to quickly locate the needed records without scanning the entire table.
Example: A table storing millions of customer records where users frequently search by
customer_id
or
2. Frequent Filtering (WHERE Clauses)
Columns that are often used in
WHERE
clauses to filter data. Indexes allow the database to quickly narrow down the result set by efficiently locating rows that match the filter criteria.Example: A table where queries often filter by
status
,date
, orcategory
.
3. Frequent Sorting (ORDER BY Clauses)
Columns that are often used in
ORDER BY
clauses for sorting data. Indexes can help the database quickly sort the data, particularly when combined with filtering.Example: An
orders
table where records are frequently sorted byorder_date
oramount
.
4. JOIN Operations
Columns that are frequently used in
JOIN
operations between multiple tables. Indexes on foreign keys and join columns improve the performance of join operations by enabling quick access to the joined records.Example: A
orders
table and acustomers
table wherecustomer_id
is used to join the two tables.
5. Unique Constraints
Columns that need to enforce unique constraints. Indexes are necessary to enforce uniqueness in a column, ensuring no duplicate values.
Example: An
users
table that must be unique for each user.
6. Foreign Key Columns
Columns that are defined as foreign keys referencing primary keys in other tables. Indexes on foreign key columns improve the performance of lookups, updates, and deletions involving the related tables.
Example: A
product_id
column in anorder_items
table that references theid
in aproducts
table.
7. Large Tables
Tables with a large number of rows where full table scans are costly.As the table grows, the performance benefit of an index becomes more significant because the cost of a full table scan increases.
Example: A
logs
table that stores system logs, which grows continuously and contains millions of records.
8. Aggregations (GROUP BY Clauses)
Columns that are frequently used in
GROUP BY
clauses to aggregate data. Indexes can improve the performance of aggregate functions by grouping records more efficiently.Example: A
sales
table where data is grouped byregion
orproduct_category
for reporting.
When Not to Use Indexes
While indexes are powerful, there are scenarios where they might not be beneficial:
- Low-Volume Tables: For small tables, the overhead of maintaining an index may outweigh the benefits, as full table scans are relatively cheap.
- Frequent Writes: Indexes can slow down
INSERT
,UPDATE
, andDELETE
operations because the index needs to be maintained along with the data. - Low-Cardinality Columns: Columns with a small number of unique values (e.g.,
gender
,status
) might not benefit much from indexing, as the index may not significantly reduce the search space. - Temporary Tables: Tables that are created and dropped frequently, especially if they are small, might not need indexing.
Technical Insights
How indexing works in the background
- Index Creation: When an index is created on a column, the database builds a separate data structure, typically a B-tree or hash table, that stores the column’s values in a sorted or hashed format along with pointers to the actual rows in the table.
- Data Changes:
Inserts: When a new row is added, the database updates the index by inserting the new value into the index structure.
- Updates: If an indexed column is updated, the index is modified to reflect the new value.
- Deletes: When a row is deleted, the corresponding entry in the index is removed.
3. Query Optimization: During a query, the database uses the index to quickly locate rows, avoiding a full table scan. This makes data retrieval much faster.
4. Maintenance: The database automatically keeps the index balanced and optimized, ensuring it remains efficient even as data changes.
Indexes operate behind the scenes, improving query speed by allowing the database to access data more efficiently
Specifics of Indexing
Are indexes dependent on database providers?
Yes, indexes can be dependent on the database provider, as different database management systems (DBMS) may implement and optimize indexes differently.
Index Types: Different databases support different types of indexes (e.g., B-tree, hash, GiST, GIN). While most relational databases support B-tree indexes, specialized index types like GIN or GiST are specific to systems like PostgreSQL.
Index Implementation: The internal implementation of how indexes are stored and managed can vary between database providers. This affects performance and behavior under certain conditions (e.g., how index maintenance is handled during large data loads).
Syntax and Features: The syntax for creating and managing indexes can differ. For example, MySQL, PostgreSQL, and SQL Server have slightly different commands and options for defining indexes (e.g., partial indexes in PostgreSQL).
Optimization and Usage: How a DBMS optimizes queries using indexes can vary. The database query planner decides when and how to use indexes, and these decisions are based on the specific DBMS’s algorithms and configurations.
While the concept of indexing is universal across databases, the specifics can depend heavily on the database provider you are using.
Practical Examples of Indexing
Consider a table Orders
, which tracks customer purchases in an e-commerce application. This table might look like this:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, OrderDate DATE, TotalAmount DECIMAL(10, 2) );
Assume that this table contains millions of records, which is common in a large-scale e-commerce environment.
Use Case: Querying Order Data
A common query might involve retrieving all orders made by a particular customer within a specific date range. For example:
SELECT * FROM Orders WHERE CustomerID = 12345 AND OrderDate BETWEEN '2023-01-01' AND '2023-12-31';
Impacts of Not Having Indexes
Without proper indexing, the database engine will perform a full table scan to satisfy this query. This means it will inspect every row in the Orders
table, leading to significant performance degradation, especially as the number of records grows.
- High CPU and I/O Usage: Scanning millions of rows consumes a lot of CPU time and I/O operations, slowing down the query execution.
- Increased Query Latency: Users will experience slower response times, which could lead to frustration and a poor user experience.
- Scalability Issues: As the table grows, the query performance will degrade even further, making the system less responsive.
Resolving the Issue with Indexing
To resolve this performance issue, you can create an index on the CustomerID
and OrderDate
columns:
CREATE INDEX idx_customer_orderdate ON Orders (CustomerID, OrderDate);
This index allows the database to quickly locate the relevant records without scanning the entire table.
Performance Comparison and Impacts
- Before Indexing: Suppose the query took 10 seconds to execute due to the full table scan. This slow performance could lead to timeouts or delays in the application.
- After Indexing: With the index in place, the query execution time might reduce to just a few milliseconds. The database engine can now use the index to jump directly to the relevant records, bypassing the need for a full table scan.
This example illustrates how proper indexing can dramatically improve database query performance. By strategically placing indexes on frequently queried columns, you can avoid full table scans, reduce resource consumption, and ensure your application remains responsive as data volumes grow.
Monitoring and Optimization
Optimizing Database Performance with Digma: Identifying Query Performance Issues
Requirements:
● Setup project using Java, Spring boot
● Install the IDE plugin.
● Instrument code using Automatic Instrumentation in the IDE, we also can add Observability to each part of our code using the Plugin.
Digma Plugin provides developers with a powerful set of features designed to identify and highlight performance issues in database queries. Proactive Insights: it notifies you of potential performance issues as you code, helping you catch and address problems early in the development cycle.
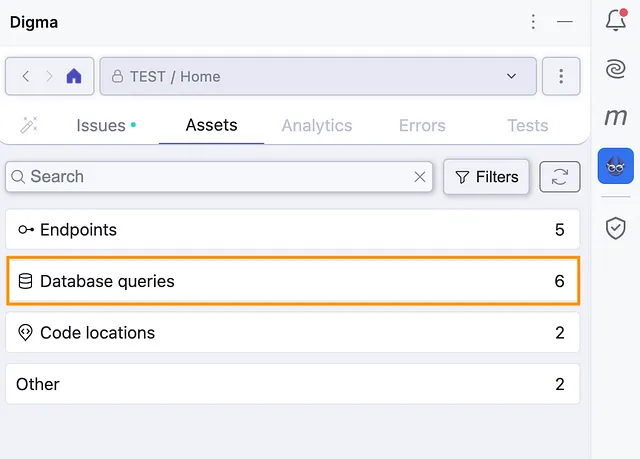
Here’s how Digma can assist in identifying performance issues in our queries.
Query Issue Detection
Digma analyzes database query traces to identify common issues and inefficiencies. It helps detect problems such as N+1 Selects queries and suboptimal bulk inserts.
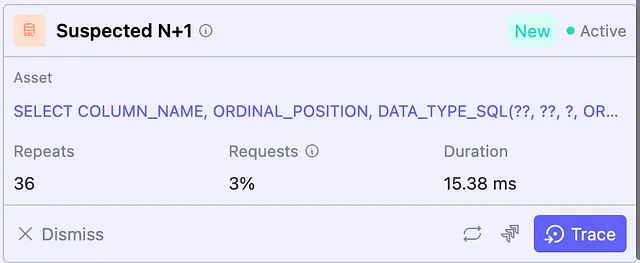
High number of queries
This feature detects and highlights endpoints within the application that are generating an unusually high number of database queries. Such behavior often indicates potential inefficiencies in the data access layer, such as excessive query abstraction or failure to leverage batch processing techniques when appropriate. Identifying and addressing these inefficiencies is crucial for maintaining optimal performance and preventing potential bottlenecks in production environments.
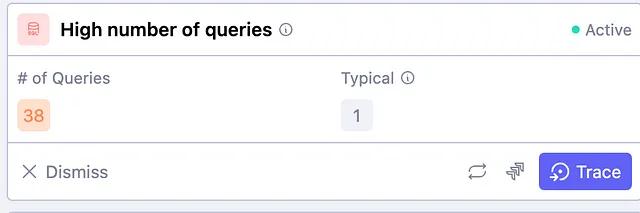
Conclusion
Indexes should be used strategically to optimize query performance. Understanding the data access patterns, query frequency, and the nature of the data itself (e.g., cardinality, size) is crucial in deciding where and when to implement indexes.
By carefully considering these conditions, you can significantly enhance the efficiency of your database operations.
Enhance Query Performance. Start your free trial now!
FAQ
- How do indexes improve database performance?
Indexes speed up data retrieval by allowing the database to quickly locate and access rows, reducing the need for full-table scans. This leads to faster query execution, especially for large datasets. - Why can too many indexes slow down database performance?
While indexes improve read performance, they add overhead to write operations likeINSERT
,UPDATE
, andDELETE
because the indexes must be updated as well. Too many indexes can lead to slower write times and increased storage costs. - What is the impact of database normalization on performance?
Normalization reduces data redundancy and improves data integrity, but it can lead to more complex queries involving multiple table joins, which may slow down performance. In some cases, denormalization (combining tables) is used to optimize read-heavy operations.