Even bad code can function. But if code isn’t clean, it can bring a development organization to its knees – Uncle Bob.
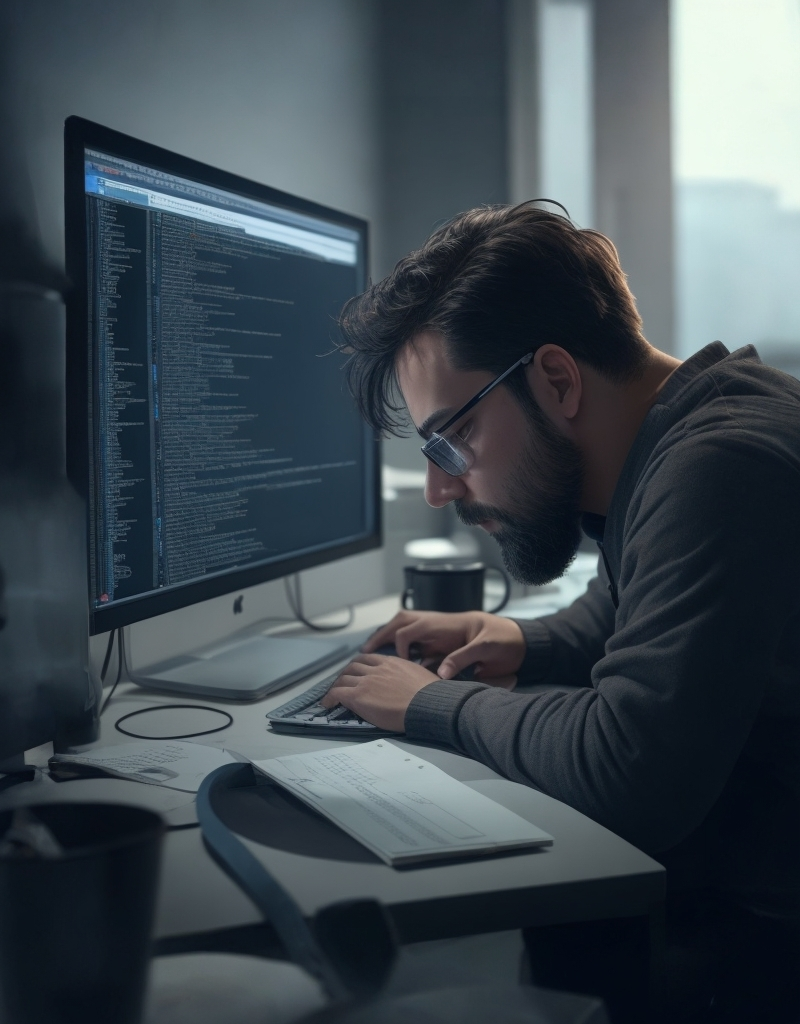
Having spent a few years in the technology industry, mostly developing software, I’ve been accustomed to the reality that most of my time is spent working with different teams and reviewing code written by other software developers. Over the years, I’ve had diverse experiences working with code written by different developers. These experiences have fundamentally reinforced my appreciation for clean code.
“Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” from Clean Code: A Handbook of Agile Software Craftsmanship (Uncle Bob).
Whether you have been a software developer for a while or just started, I’m confident that you can relate to the experience and effort required when inheriting a new codebase. Inheriting a codebase often involves familiarizing yourself with the code, its structure, functionality, programming language, libraries, framework, and any other technology used.
Navigating unfamiliar code or code you wrote a while ago can be daunting. Whether you want to fix bugs, enhance functionality, improve performance, or join an ongoing project, the time and effort required depends on the state of the code.
Code written with clean Java code principles can save you countless hours and frustrations. On the other hand, when working with messy code, you’re likely to spend most of your time deciphering tangled logic, uncommented code, and poorly named variables.
Best Practices for writing clean Java code
Best Practices for writing clean Java code.
What is clean Java code and its benefits?
How to write clean Java code.
1. Use a Standard Project Structure.
2. Stick to Java Naming Convention.
3. Readability over Reusability.
4. Lint your code with Static and Dynamic analysis tools.
5. Use Meaningful comments and documentation.
6. Use consistent and proper code formatting: whitespaces and indentation.
7. Restrict the number of method parameters.
8. Leverage Unit Tests and Test Driven Development (TDD).
9. SOLID principles.
10. Keep It Simple, Studio (KISS), and Don’t Repeat Yourself (DRY) principle.
11. The Source Files Structure.
12. Avoid Hard coding values.
What is clean Java code and its benefits?
Clean code is the practice of writing straightforward, readable, testable, and easy-to-understand code. Other features of clean code include adherence to good conventions and best practices that promote expressiveness, conciseness, organization, and maintainability. Clean code should also be free from bugs, unjustifiable complexity, code smells, and redundant code.
Robert C. Martin, popularly known as Uncle Bob, has written extensively on the subject of clean code. Programmers of all levels of experience who desire to write clean code can benefit from his book on clean code, articles, and a series of talks. In his book, he says,
“ Even bad code can function. But if code isn’t clean, it can bring a development organization to its knees. Every year, countless hours and significant resources are lost because of poorly written code. But it doesn’t have to be that way ” from Clean Code: A Handbook of Agile Software Craftsmanship.
The importance of writing clean Java code cannot be overstated. Some benefits that can be immediately realized from clean code include:
- Maintainability – Clean code is easy to modify and update.
- Debugging – Clean code is less prone to errors. It is also easier to isolate and fix issues within the code.
- Scalability – Clean code is modular, reusable, and accommodative of future changes.
- Collaboration – Clean code allows teammates to understand each other’s code.
- Documentation – Clan code is self-explanatory and reduces the need for excessive comments.
- Efficiency – Clean code removes code duplication and unnecessary complexity, which improves performance.
- Readability – Clean code is easy to read, reduces confusion, and improves maintainability.
How to write clean Java code
Java is still a popular programming language. Since its an established language, legacy Java codebases are still critical in running important business software and infrastructure developed over a decade ago and are still in use by thousands of users.
Due to the longevity of Java codebases, it’s important to write clean Java code that can be easily maintained by developers that come after you. Here are the best practices to help you write clean Java code.
1. Use a Standard Project Structure
Project structure outlines how to arrange various components in your project, such as Java source files, test files, documentation files, build files, and configuration files. A clear project structure makes it easy to understand, navigate and modify a project codebase. On the other hand, a poor project structure can cause confusion, especially when working with projects with many files.
Although Java doesn’t enforce a specific project structure, build tools such as Maven suggests a project structure you can follow.
src ├── main │ ├── java Application/Library sources │ ├── resources Application/Library resources │ ├── filters Resource filter files │ └── webapp Web application sources │ └── test ├── java Test sources ├── resources Test resources └── filters Test resource filter files
2. Stick to Java Naming Convention
Java naming conventions are a set of rules that dictate how Java developers should name identifiers. The Java Specification Document includes rules for naming variables, packages, classes, and methods. These naming conventions allow developers to keep things in order when writing code. Good naming improves code readability, consistency, and maintainability.
Some of the Java naming conventions include:
- Class and interface names should be nouns and have the first letter capitalized.
- Method names should be verbs.
- Variable names should be short and meaningful.
- Package names should be lowercase.
- Constant names should be capitalized.
package com.example.project; public class Person { private String firstName; private String lastName; public Person(String firstName, String lastName) { this.firstName = firstName; this.lastName = lastName; } public String getFullName() { return firstName + " " + lastName; } public static final int MAX_AGE = 100; public boolean hasValidName() { return firstName != null && lastName != null; } }
You can find more information about Java naming conventions here.
3. Readability over Reusability
Reusability is one of the most advocated concepts in software development. It lessens development time and reduces the effort required to maintain software when developers understand the reusable components very well.
While the concept of reusability sounds great and has many benefits, it also has many potential pitfalls, especially when working on an unfamiliar codebase. When working with large applications, code reusability can reduce readability, usability, and maintainability if a proper design is not in place. Code reusability affects the readability of code when it makes it difficult to understand the logical flow of the code without tracing execution.
Poor code readability makes debugging difficult and increases the effort required to maintain the codebase. This can be challenging, especially when trying to onboard new developers into your project. Therefore, as you develop software, ensure that you don’t prioritize reusability over readability.
4. Lint your code with Static and Dynamic analysis tools
Static and dynamic code analysis tools complement each other. Both dynamic and static analysis tools can help in writing clean Java code. Static analysis tools allow you to inspect your application source code and ensure adherence to coding standards, spot vulnerabilities, and detect bugs during development.
On the other hand, dynamic analysis allows you to test your application at runtime. It allows you to measure your application’s performance, behavior, and functionality and identify runtime errors, memory leaks, and resource consumption reducing the chances of running into issues in production.
Digma is a runtime linter that lets developers quickly identify risky code, potential errors, and bottlenecks in complex codebases. Digma leverages OpenTelemetry behind the scene to collect data such as traces, logs, and metrics. Once the data is collected, Digma analyzes it to detect meaningful insights about the code. It looks for regressions, anomalies, code smells, or other patterns that can be useful for knowing about the code, allowing you to push clean Java code to production.
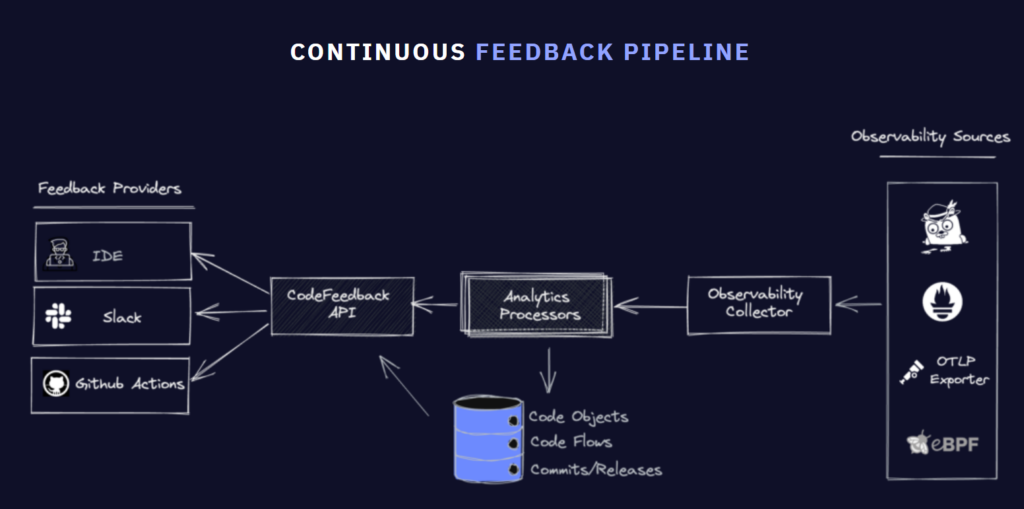
5. Use Meaningful comments and documentation
Obsessive commenting is something I struggled with, especially early on in my software development career. This is something that most developers struggle with. Improper use of comments indicates that your code is a symptom of bad programming.
Proper use of comments and documentation can serve an important role in writing clean Java code. While code should be readable and self-explanatory, sometimes it’s impossible to avoid complex logic. However, using strategic comments within the code, you can explain the logic behind some parts of the code that are not straightforward.
In Java, developers can leverage two types of comments documentation comments and implementation comments. Documentation comments target codebase users, while implementation comments are meant for developers working on the codebase.
/** * This class represents a RESTful controller for managing user resources. * It provides endpoints for creating, retrieving, updating, and deleting users. */ @RestController @RequestMapping("/api/users") public class UserController { /** * Retrieves a user by ID. * * @param id The ID of the user to retrieve. * @return The user with the specified ID. */ @GetMapping("/{id}") public ResponseEntity<User> getUserById(@PathVariable("id") Long id) { // Implementation omitted for brevity } /** * Creates a new user. * * @param user The user object to create. * @return The created user. */ @PostMapping public ResponseEntity<User> createUser(@RequestBody User user) { // Implementation goes here } //Rest of the code
6. Use consistent and proper code formatting: whitespaces and indentation
Code formatting may not seem like a big issue when you’re working on a personal project whose code may never be maintained by another developer. However, consistent code formatting and style are critical when working with a team of other developers.
Maintaining a consistent formatting and coding style in your team and codebase is important if you wish to write clean Java code as a team. Whitespace and indentation are essential in maintaining a consistent coding style.
Good usage of whitespace between operators, commas, and around flow statements enhances code readability. For instance, you can organize code into logical groups using whitespaces, enhancing readability and visual clarity.
Indentation refers to using tabs or spaces within loops, methods, and control structures. Although there is no enforced convention for code indention in Java, you can choose to adopt popular convection and use it consistently.
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class MyApplication { public static void main(String[] args) { SpringApplication.run(MyApplication.class, args); } // Rest of the code goes here // ... }
7. Restrict the number of method parameters
Parameters are necessary when working with methods. However, care should be taken to avoid using too many parameters in one method. Too many parameters may indicate that your method is addressing more than one concern and violates the single responsibility principle.
Too many method parameters make your code less readable since keeping track of their types and meanings is difficult. To write clean Java code, you should: limit the number of method parameters and use objects or data structures instead of individual parameters or group-related parameters into objects.
Here is an example of a Java method with too many method parameters.
public void processOrder(String customerName, String shippingAddress, String billingAddress, String productName, int quantity, double price, boolean isExpressShipping) { // Method implementation }
Here is how we can refactor the code above by grouping related parameters into an object to improve readability.
public class Order { private String customerName; private String shippingAddress; private String billingAddress; private String productName; private int quantity; private double price; private boolean isExpressShipping; // Constructors, getters, and setters // Other methods related to an order } public void processOrder(Order order) { // Method implementation }
8. Leverage Unit Tests and Test Driven Development (TDD)
Unit testing and TDD are very common practices in software development. Unit testing involves writing tests for individual functions, methods, and classes to ensure that they work in isolation. TDD is also a popular development practice that involves writing tests before code.
Both Unit tests and a TDD approach can propel your efforts toward writing clean Java code. Unit tests allow you to verify correctness, detect bugs early, and write more modular code. TDD provides immediate feedback and increases your confidence in writing reliable and maintainable code.
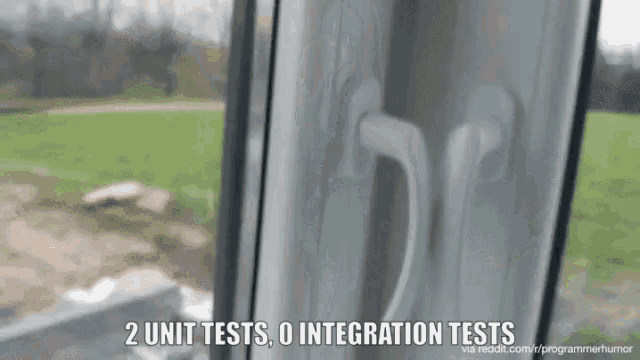
Source: Twitter
9. SOLID principles
The SOLID principles by Robert C. Martin (Uncle Bob) are very popular; every developer should know them. These principles can also help you write clean, maintainable, and scalable code.
Let’s discuss how each of the principles in the SOLID acronym can help you write clean Java code.
Single Responsibility Principle (SRS): The single responsibility principle states that a class should only have one responsibility. Following the SRS principle guarantees that we write concise, readable, and maintainable code.
Open-closed principle (OCP): The OCP states that classes should be open for extension but closed for modification except when fixing bugs. This principle allows you to add new features without introducing bugs or breaking existing functionality. In Java, you can use interfaces or abstract classes to extend the functionality of existing classes.
Liskov substitution principle (LSP): The LSP principle states that we should be able to use superclasses with their respective subclasses interchangeably without breaking the functionality of our program. Using this principle allows you to use inheritance correctly and write decoupled clean Java code.
Interface Segregation Principle: States that we should opt for smaller focused interfaces instead of large monolithic ones. Using this principle allows us to write modular and clean Java code where the implementing classes focus only on methods that concern them.
Dependency Inversion Principle (DIP): This principle emphasizes loose coupling, guaranteeing that components such as classes only depend on abstractions and not on their concrete implementations. DIP helps us enforce clean Java code using Inversion of Control (IoC) and dependency injection.
10. Keep It Simple, Studio (KISS), and Don’t Repeat Yourself (DRY) principle
KISS and DRY are very fundamental concepts in software development and can help you write clean Java code. The DRY principle states that as a developer, you should ensure that your code is not duplicated multiple times across the system. Eliminating code duplication improves your code maintainability and makes finding and fixing bugs easier.
The KISS principle emphasizes that we should strive to keep the design and development of the software we build simple and straightforward. By following this principle, you can avoid unnecessary complexity in your code and instead opt to write simple and understandable code.
The KISS principle promotes the maintainability of your code and makes it more readable. Maintainable and readable code improves collaboration and eases the onboarding of developers into the project.
11. The Source Files Structure
A typical source file in Java contains different elements that are key in running any Java program. To maintain the readability of your code, you should enforce a consistent source file structure. Although there is no one-size-fits approach to structuring your source file, there are popular style guides that you can follow.
Normally a typical ordering of a source file structure in Java begins with package statements followed by static and non-static import statements, preceded by one primary top-level class.
// Class variables private static int count; private String name; // Instance variables private int age; private List<String> hobbies; // Constructors public MyClass() { // Constructor implementation } public MyClass(String name, int age) { // Constructor implementation } // Methods public void setName(String name) { // Method implementation } public String getName() { // Method implementation } public void addHobby(String hobby) { // Method implementation } // Other methods } // Additional classes (if any) class MyStaticClass { // Class implementation }
12. Avoid Hard coding values
Hardcoding refers to embedding values directly into your program’s source code instead of using variables. Altering the source code of your program is the only way to change values hardcoded into your programs. Hardcoded values limit reusability and testability and can also lead to duplication and undesired behavior from your program.
To improve code reusability, testability, and maintainability, which are key features of clean Java code, it is important that you avoid hard coding values in your program source code. Instead, replace hard-coded values with abstractions such as constant variables or enums.
Here’s an example of a Java program with hardcoded values.
@RestController public class HelloWorldController { @GetMapping("/hello") public String sayHello() { return "Hello, World!"; } @GetMapping("/user") public String getUser() { // hardcode values String username = "John"; int age = 30; return "Username: " + username + ", Age: " + age; } // Other controller methods }
Conclusion
Writing clean Java code is key to developing high-quality software.
In this article, we have shared some of the best and most common practices that can help you write clean Java code. However, it is important to note that this isn’t a definitive list of what it takes to write clean Java code. Other key factors that contribute to writing clean Java code include the culture, tools available, and goals of the team you’re working with. You can write readable, testable, extensible, and modular code by embracing these principles.
FAQ
What is clean code?
Clean code refers to readable, and maintainable code that is written based on best practices and conventions. It is code that is easy to understand, modify, and extend by both the original author and other developers. who inherited the code.
Why is it important to write clean Java code?
Clean code improves readability, enhances collaboration, reduces the chances of introducing bugs, and makes maintainability easier.
Are there tools that can help in writing clean Java code?
There are many tools that aid the process of writing clean Java code. Some include static code analysis tools such as SonarQube, FindBugs, and Digma. IDEs such as IntelliJ and Eclipse can also be helpful.
Which resources can I use to learn more about writing clean Java code?
Plenty of blogs and online tutorials exist, such as Baeldung. I would also recommend books such as “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin and “Effective Java” by Joshua Bloch.